JS Panorama
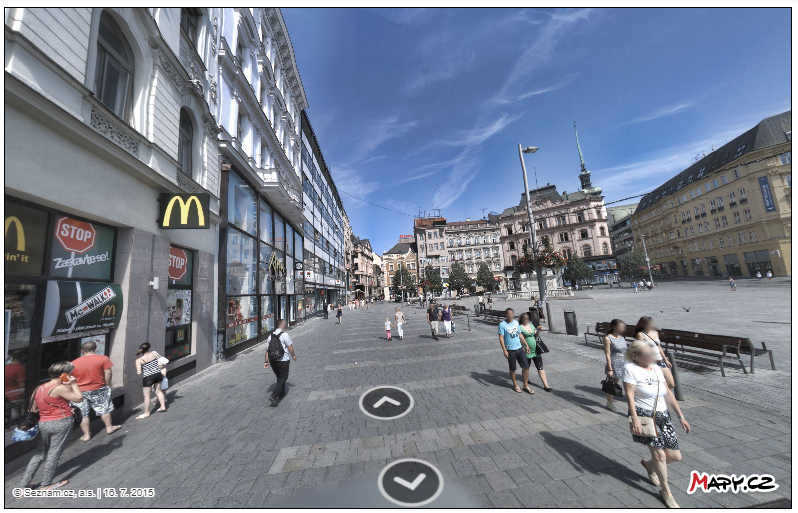
JavaScript component for displaying panoramic images from all over the Czech Republic.
This “panorama viewer” displays spherical images on your website and allows users to look around or move to adjacent images.
The component takes care of loading necessary data via REST API.
For specific examples, see Panorama tutorials.
The images were taken in various parts of the country and in different years. For licensing reasons, only images up to 2019 are currently available via the API.
Panorama Control
Users can navigate the panorama using the mouse or keyboard (left/right arrows or “a” and “d” keys).
If navigation between snapshots is enabled (showNavigation:true), users can switch between snapshots using the mouse (by clicking on navigation arrows or other snapshot areas) or the keyboard (forward/backward arrows or “w” and “s” keys).
Panorama Consumption
A panorama (one sphere) is not downloaded as a single large photo but is composed of individual panorama tiles. The usual size of the entire panorama is 16×8 tiles.
The JS viewer takes care of downloading the necessary tiles. It doesn’t download the entire sphere but only the tiles that are within the displayed view.
The consumption of panorama tiles is then charged according to the REST API pricing.
Using the Library
To use the panorama, you need to add this script to the header.
<script type="text/javascript" src='https://api.mapy.cz/js/panorama/v1/panorama.js'></script>
With this script, you have access to the Panorama class, which exposes a single panoramaFromPosition() function.
Function panoramaFromPosition()
This function is used for displaying the panorama at specific coordinates. It searches for the nearest panorama to the given coordinates within a specified radius.
Input Parameters
parent | parent HTML element for attaching the panorama |
lon, lat | coordinates around which the nearest panorama is searched, up to the specified radius (see the radius parameter) |
apiKey | API key for REST API |
Optional Parameters | |
radius | Maximum radius for finding the panorama. In meters. default value: 50 m |
yaw | Orientation relative to the north Possible values: “auto” – default value, orientation follows the direction of the panorama “point” – orientation towards the input coordinates number – custom value within the range 0 – 2 * Math.PI (0 – north) |
pitch | Tilt (- up, + down) Possible values: +-Math.PI default value: 0 |
fov | Horizontal field of view (in fact zoom) Possible values: Math.PI / 2 – Math.PI/20 default value: 1.2 |
showNavigation | true/false – enables or disables the option to move to neighboring snapshots default value: false |
lang | language (used for tooltips, error messages, etc.) Possible values: cs, de, el, en, es, fr, it, nl, pl, pt, ru, sk, tr, uk default value: cs |
Return Values
The function returns an IOutput object, which contains the following keys:
Panorama Information | |
info.lon, info.lat | Information about the actual location of the photographed place |
info.date | Date of taking the snapshot in the format YYYY-MM-DD hh:mm:ss |
Status Information | |
errorCode | “NONE” | “PANORAMA_NOT_FOUND” | “MISSING_API_KEY” |
error | Description of the error |
Functions | |
destroy function | A function that removes the panorama |
addListener, removeListener functions | event tracking for panorama view change “pano-view” and panorama place change “pano-place” (see example) |
setCamera, getCamera functions | functions for setting/reading the current panorama view |
interface ICreatePanoFromPositionOpts {
/** Parent element */
parent: HTMLElement;
/** Wgs84 longitude coordinate */
lon: number;
/** Wgs84 latitude coordinate */
lat: number;
/** API key */
apiKey: string;
/** Default pano view - yaw - "auto", "point" - direction to point, number - custom value <0;2 * Math.PI> [rad] */
yaw?: "auto" | "point" | number;
/** Default pano view - pitch */
pitch?: number;
/** Horizontal field of view */
fov?: number;
/** Search area radius [m] */
radius?: number;
/** Supported langs: cs, de, el, en, es, fr, it, nl, pl, pt, ru, sk, tr, uk */
lang?: TLANGS;
/** Show navigations - neighbors, click mask */
showNavigation?: boolean;
/** Hide errors output */
hideErrors?: boolean;
}
interface ICreatePanoFromPositionOutput {
/** Panorama meta information */
info?: {
/** Pano wgs84 longitude coordinate */
lon: number;
/** Pano wgs84 latitude coordinate */
lat: number;
/** Create date YYYY-MM-DD hh:mm:ss*/
date: string;
};
/** Error message */
error: string;
/** Error code */
errorCode: "NONE" | "PANORAMA_NOT_FOUND" | "MISSING_API_KEY" | "WRONG_API_KEY";
/** Add listener */
addListener: (name: T, callback: (data: IEvent[T]) => void) => void;
/** Remove listener */
removeListener: (name: T, callback: (data: IEvent[T]) => void) => void;
/** Get panorama camera */
getCamera: () => ICamera;
/** Set panorama camera */
setCamera: (camera: ICamera) => void;
/** Destroy panorama */
destroy: () => void;
}
interface ICamera {
yaw: number;
pitch: number;
fov: number;
}
Tracking Rotation and Translation
You can connect to events that are triggered when the view is changed or when the panorama moves to another location.
// add listener - pano view change
panoData.addListener("pano-view", viewData => console.log(["view data", viewData]));
// add listener - pano change place
panoData.addListener("pano-place", placeData => console.log(["place data", placeData]));
Function panoramaExists()
This function checks if a panorama is available at the given location. Input parameters and return values are similar to the panoramaFromPosition() function.
interface IPanoramaExistsOpts {
/** Wgs84 longitude coordinate */
lon: number;
/** Wgs84 latitude coordinate */
lat: number;
/** API key */
apiKey: string;
/** Search area radius [m] */
radius?: number;
}
interface IPanoramaExistsOutput {
/** Panorama meta information */
info?: {
/** Pano wgs84 longitude coordinate */
lon: number;
/** Pano wgs84 latitude coordinate */
lat: number;
/** Create date YYYY-MM-DD hh:mm:ss*/
date: string;
};
/** Panorama exists? */
exists: boolean;
}